Advanced Field Symbol Usage in SAP ABAP: Modern Techniques for Developers
Discover advanced and modern techniques for using field symbols in SAP ABAP. Learn how to enhance performance and write cleaner, more efficient code.
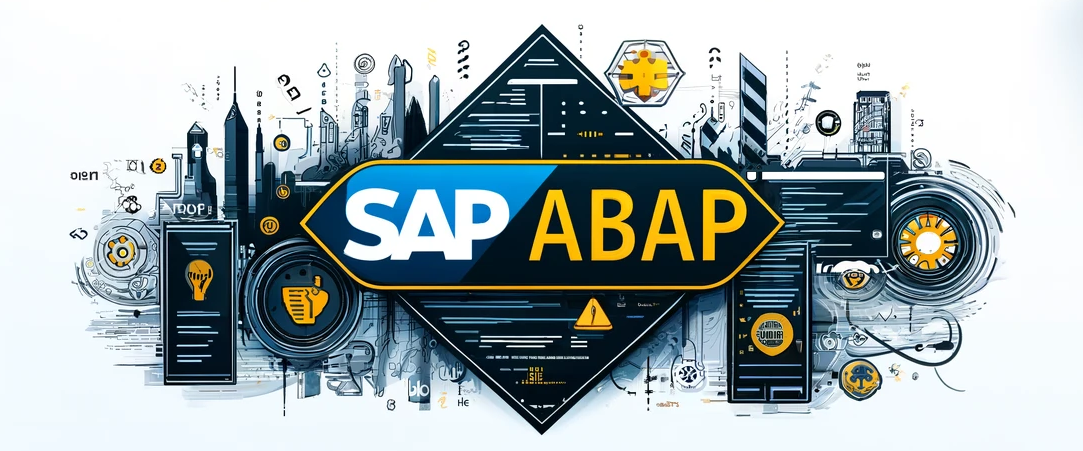
Advanced Field Symbol Usage in SAP ABAP: Modern Techniques for Developers
As SAP ABAP developers, we often encounter situations where we need to manipulate data dynamically. Field symbols in ABAP provide a powerful way to achieve this by allowing us to work with data references dynamically. This blog post delves into advanced techniques for using field symbols, providing practical examples and best practices for modern SAP ABAP development.
What are Field Symbols?
Field symbols are placeholder variables that allow us to reference other data objects dynamically. They are akin to pointers in other programming languages like C or C++. By using field symbols, we can achieve greater flexibility and efficiency in our ABAP code.
Declaring Field Symbols
Field symbols are declared using the FIELD-SYMBOLS
statement. Here’s a basic example:
FIELD-SYMBOLS <fs_field> TYPE any.
In this example, <fs_field>
is a field symbol that can point to any data object. We use the ASSIGN
statement to assign a data object to the field symbol:
DATA lv_value TYPE i VALUE 10.
ASSIGN lv_value TO <fs_field>.
Advanced Techniques
1. Dynamic Data Manipulation
Field symbols allow us to manipulate data structures dynamically. Consider the following example where we dynamically modify the contents of an internal table:
DATA: lt_table TYPE TABLE OF string,
lv_index TYPE i.
FIELD-SYMBOLS <fs_row> TYPE string.
APPEND 'ABAP' TO lt_table.
APPEND 'Field' TO lt_table.
APPEND 'Symbols' TO lt_table.
lv_index = 2.
READ TABLE lt_table INDEX lv_index ASSIGNING <fs_row>.
IF sy-subrc = 0.
<fs_row> = 'Modified'.
ENDIF.
In this example, we read the second row of the internal table and modify its content dynamically using a field symbol.
2. Dynamic Structure Handling
Field symbols are particularly useful when working with dynamic structures. The following example demonstrates how to dynamically assign components of a structure:
DATA: BEGIN OF ls_structure,
comp1 TYPE i,
comp2 TYPE string,
END OF ls_structure.
FIELD-SYMBOLS <fs_component> TYPE any.
ASSIGN COMPONENT 'COMP2' OF STRUCTURE ls_structure TO <fs_component>.
IF sy-subrc = 0.
<fs_component> = 'Dynamic Assignment'.
ENDIF.
Here, we dynamically assign and modify the COMP2
component of the structure ls_structure
using a field symbol.
3. Enhancing Performance
Field symbols can enhance the performance of your ABAP programs by reducing the need for deep copies of data. Instead of copying large data sets, you can use field symbols to work with references directly. This is particularly beneficial when working with large internal tables.
4. Working with Dynamic Data References
Field symbols can be used to reference dynamic data objects. This can be particularly useful when dealing with data of unknown types at runtime:
DATA: lr_data TYPE REF TO data,
lv_length TYPE i.
FIELD-SYMBOLS <fs_data> TYPE any.
CREATE DATA lr_data TYPE string.
ASSIGN lr_data->* TO <fs_data>.
<fs_data> = 'Dynamic String'.
lv_length = strlen( <fs_data> ).
In this example, we create a data reference to a string and then use a field symbol to manipulate the data.
5. Handling Internal Tables with Header Lines
Field symbols can simplify the manipulation of internal tables with header lines. Consider the following example:
DATA: lt_table TYPE TABLE OF string WITH HEADER LINE.
FIELD-SYMBOLS <fs_row> TYPE string.
APPEND 'Header Line 1' TO lt_table.
APPEND 'Header Line 2' TO lt_table.
LOOP AT lt_table ASSIGNING <fs_row>.
WRITE: / <fs_row>.
ENDLOOP.
In this example, we loop through an internal table with header lines and print each row using a field symbol.
6. Complex Dynamic Structures
Field symbols can be used to navigate and manipulate complex dynamic structures. Here’s an advanced example:
DATA: BEGIN OF ls_dynamic,
part1 TYPE i,
part2 TYPE string,
END OF ls_dynamic.
FIELD-SYMBOLS <fs_dynamic> TYPE any.
ASSIGN ls_dynamic TO <fs_dynamic>.
ASSIGN COMPONENT 'PART2' OF STRUCTURE <fs_dynamic> TO <fs_dynamic>.
IF sy-subrc = 0.
<fs_dynamic> = 'Updated Part2'.
ENDIF.
In this example, we dynamically assign and update a component of a complex structure.
Best Practices
While field symbols are powerful, they should be used judiciously. Here are some best practices to consider:
- Ensure proper error handling when using the
ASSIGN
statement to avoid runtime errors. - Use descriptive names for field symbols to improve code readability.
- Avoid excessive use of field symbols, as they can make code harder to debug and maintain.
Conclusion
Field symbols are a powerful feature in SAP ABAP that can greatly enhance the flexibility and performance of your code. By mastering advanced techniques and following best practices, you can leverage field symbols to create dynamic and efficient ABAP applications.
Happy coding!